If you work on WordPress websites, here is a tip you might find helpful.
It requires adding code to the functions.php file.
Tested with WordPress 5.
How to add links to the WordPress admin bar
Depending on the WordPress project you are working on, it can be very useful to have a quick access to specific links from the admin bar, for instance in case of very frequent accesses to the Customize and Plugins pages, a case that we will deal with in this tutorial.
The code example below allows you to add two links to the WordPress admin bar : A “Customize” link, and a “Plugins” link, exclusively for users having the WordPress Administrator role.
It will make so that :
- Both links will appear on back-end pages.
- The Customize link won’t appear on front-end pages.
By default, WordPress already displays a Customize link in admin bar on front-end pages for users whose roles allow it.
You can use the following code snippet as is : Just copy-paste it to the end of contents of your theme’s functions.php file.
$user = wp_get_current_user();
if ( in_array("administrator", $user->roles) ) { // Checking if user has Administrator role
if (is_admin()) { // Checking if user is in back-end
add_action( 'admin_bar_menu', 'my_wp__admin_bar_link_Customize', 100 );
function my_wp__admin_bar_link_Customize( $admin_bar ) {
$admin_bar->add_menu( array(
'id' => 'wp-admin-bar-link-customize',
'title' => __('Customize'),
'href' => home_url() . '/wp-admin/customize.php',
));
}
}
add_action( 'admin_bar_menu', 'my_wp__admin_bar_link_Plugins', 100 );
function my_wp__admin_bar_link_Plugins( $admin_bar ) {
$admin_bar->add_menu( array(
'id' => 'wp-admin-bar-link-plugins',
'title' => __('Plugins'),
'href' => home_url() . '/wp-admin/plugins.php',
));
}
}
The code above opens links in the same web browser’s tab.
Here’s a variant for opening links in a new tab :
$user = wp_get_current_user();
if ( in_array("administrator", $user->roles) ) { // Checking if user has Administrator role
if (is_admin()) { // Checking if user is in back-end
add_action( 'admin_bar_menu', 'my_wp__admin_bar_link_Customize', 100 );
function my_wp__admin_bar_link_Customize( $admin_bar ) {
$admin_bar->add_menu( array(
'id' => 'wp-admin-bar-link-customize',
'title' => __('Customize'),
'href' => home_url() . '/wp-admin/customize.php',
'meta' => array(
'target' => '_blank',
),
));
}
}
add_action( 'admin_bar_menu', 'my_wp__admin_bar_link_Plugins', 100 );
function my_wp__admin_bar_link_Plugins( $admin_bar ) {
$admin_bar->add_menu( array(
'id' => 'wp-admin-bar-link-plugins',
'title' => __('Plugins'),
'href' => home_url() . '/wp-admin/plugins.php',
'meta' => array(
'target' => '_blank',
),
));
}
}
In these examples, the code checks that the user has Administrator role (via the ‘administrator’ slug).
Let’s say that you granted plugins updating and theme customizing capabilities to the Editor role via the Members WordPress plugin (by going to Members > Roles > Editor, and checking “Grant” checkboxes regarding “Plugins > Activate Plugins”, “Plugins > Update Plugins”, and “Appearence > Edit Theme Options”), and that you want to display the admin bar “Customize” and “Plugins” links specifically for both Administrator and Editor roles.
A simple way to do this is to use the following php function, which checks if a particular user has one or more roles :
(the code goes in functions.php )
/**
* Checks if a particular user has one or more roles.
*
* Returns true on first matching role. Returns false if no roles match.
*
* @uses get_userdata()
* @uses wp_get_current_user()
*
* @param array|string $roles Role name (or array of names).
* @param int $user_id (Optional) The ID of a user. Defaults to the current user.
* @return bool
*/
function check_user_roles($roles, $user_id = null) {
if ( is_numeric( $user_id ) ) {
$user = get_userdata( $user_id );
}
else {
$user = wp_get_current_user();
}
if ( empty( $user ) ) {
return false;
}
$user_roles = (array) $user->roles;
foreach ( (array) $roles as $role ) {
if ( in_array( $role, $user_roles ) ) {
return true;
}
}
return false;
}
Then you just have to replace :
if ( in_array("administrator", $user->roles) ) { // Checking if user has Administrator role
With :
if ( check_user_roles( array("administrator", "editor") ) ) { // Checking if user has Administrator or Editor role
Note the array(“administrator”, “editor”) part : This is a php array that we pass as parameter to the check_user_roles() php function. This array contains two php strings corresponding to WordPress user’s role slugs : “administrator” and “editor”.
Here are the main WordPress user roles and the related slugs :
- Administrator (slug: ‘administrator’) – Has access to all the administration features within a single site.
- Editor (slug: ‘editor’) – Can publish and manage posts including the posts of other users.
- Author (slug: ‘author’) – Can publish and manage their own posts.
- Contributor (slug: ‘contributor’) – Can write and manage their own posts but cannot publish them.
- Subscriber (slug: ‘subscriber’) – Can only manage their profile.
Official documentation :
WordPress Plugin Handbook – Hooks
add_action WordPress php function
WordPress add_menu method reference
Best Regards.
Franck Mallouk, aka Dj Franck Goss
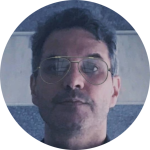